mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-02-09 21:21:39 +02:00
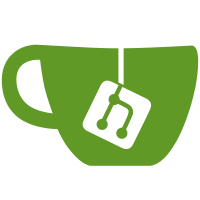
The PowerPC CPU has bits in MSR (DR and IR) which control whether addresses are translated. We should respect these instead of mixing physical addresses and translated addresses into the same address space. This is mostly mass-renaming calls to memory accesses APIs from places which expect address translation to use a different version from those which do not expect address translation. This does very little on its own, but it's the first step to a correct BAT implementation.
72 lines
1.2 KiB
C++
72 lines
1.2 KiB
C++
// Copyright 2013 Dolphin Emulator Project
|
|
// Licensed under GPLv2
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
|
|
class IniFile;
|
|
|
|
namespace PatchEngine
|
|
{
|
|
|
|
enum PatchType
|
|
{
|
|
PATCH_8BIT,
|
|
PATCH_16BIT,
|
|
PATCH_32BIT,
|
|
};
|
|
|
|
extern const char *PatchTypeStrings[];
|
|
|
|
struct PatchEntry
|
|
{
|
|
PatchEntry() {}
|
|
PatchEntry(PatchType _t, u32 _addr, u32 _value) : type(_t), address(_addr), value(_value) {}
|
|
PatchType type;
|
|
u32 address;
|
|
u32 value;
|
|
};
|
|
|
|
struct Patch
|
|
{
|
|
std::string name;
|
|
std::vector<PatchEntry> entries;
|
|
bool active;
|
|
bool user_defined; // False if this code is shipped with Dolphin.
|
|
};
|
|
|
|
int GetSpeedhackCycles(const u32 addr);
|
|
void LoadPatchSection(const std::string& section, std::vector<Patch> &patches,
|
|
IniFile &globalIni, IniFile &localIni);
|
|
void LoadPatches();
|
|
void ApplyFramePatches();
|
|
void Shutdown();
|
|
|
|
inline int GetPatchTypeCharLength(PatchType type)
|
|
{
|
|
int size = 8;
|
|
switch (type)
|
|
{
|
|
case PatchEngine::PATCH_8BIT:
|
|
size = 2;
|
|
break;
|
|
|
|
case PatchEngine::PATCH_16BIT:
|
|
size = 4;
|
|
break;
|
|
|
|
case PatchEngine::PATCH_32BIT:
|
|
size = 8;
|
|
break;
|
|
}
|
|
return size;
|
|
}
|
|
|
|
} // namespace
|
|
extern std::vector<PatchEngine::Patch> onFrame;
|